Recent Posts
-
Eyeshadow Cream: The Must-Have, Top-Rated, Bestselling Formula.
•
Eyeshadow Cream: The Must-Have, Top-Rated, Bestselling Formula Ladies and gentlemen, beauty aficionados, makeup mavens, prepare to have your eyeshadow game revolutionized! Forget everything you thought you knew about powdery pigments and fall-out frustration. ZenaMakeup proudly presents the eyeshadow cream that’s not just making waves, it’s creating a tidal wave of…
-
The Bestselling Eyeshadow Cream for Your Most Stunning Eyes.
•
The Bestselling Eyeshadow Cream for Your Most Stunning Eyes: Prepare to Be Mesmerized!
Alright, beauty aficionados, makeup mavens, and curious cats alike! Gather ’round, because I, your friendly neighborhood Product Manager at ZenaMakeup, am about to unveil a secret so dazzling, so transformative, it’ll make your peepers pop like…
-
Why This Eyeshadow Cream is Our All-Time Bestseller.
•
Why This Eyeshadow Cream is Our All-Time Bestseller (and Why You Need It in Your Life, Like, Yesterday!) Alright, makeup mavens, beauty buffs, and eyeshadow enthusiasts (and even you lovely folks who are eyeshadow-curious!), gather ‘round! Let’s talk about something truly magical, something that has transformed the makeup bags of…
Customer is paramount
We put our customers first
Putting you, our valued customer, first is the core of everything we do. We are dedicated to understanding your unique beauty needs and aspirations, always listening to your feedback.
This guides us in carefully selecting premium ingredients and developing innovative, effective formulas designed specifically for you. We provide personalized service, ensuring every interaction offers a seamless and delightful experience. Your satisfaction, radiant beauty, and unwavering confidence are our greatest rewards.
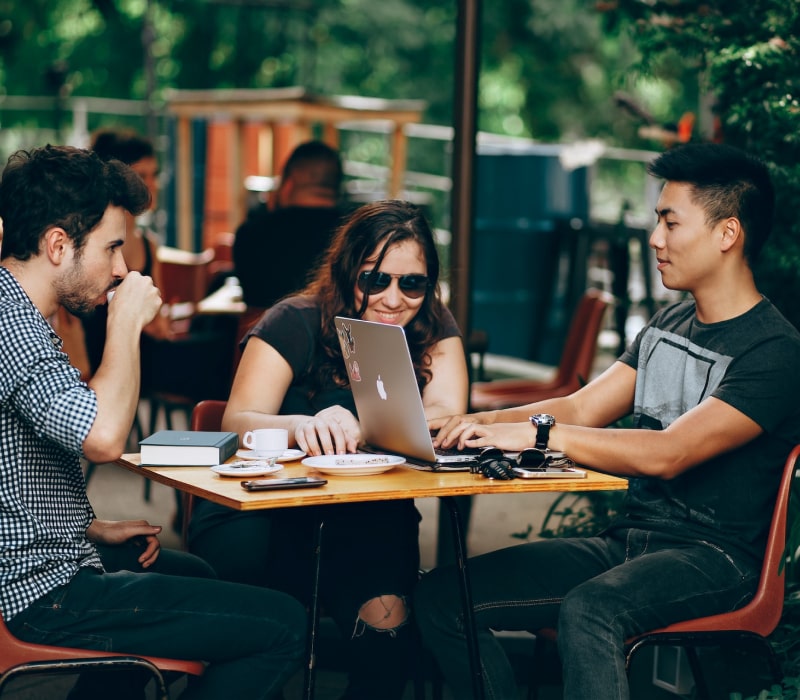