list
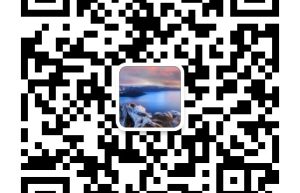
UML demonstration of Java serialization 82Set, Collection, List, and Map
1. UML Demonstration Collection Collection Collection Inheritance Structure Diagram 2. Set collection 1. Characteristics of List storage elements: ordered and repeatable. In order, what is the order in which they are stored and what order they are taken out. 2. Characteristics of Set storage elements: unordered and non-repeatable. When they are stored in the same order, when they are taken out, they may not be in the original order. 3.SortedSet features Characteristics of storing elements: Unordered and non-repeatable, but the stored elements can be automatically sorted according to the size of the elements. 3. Introduction to the underlying data structure of commonly used collection classes 1. The bottom layer of ArrayList uses an array to store elements, so the ArrayList collection is suitable for querying, but not suitable for frequent random additions and deletions of elements. 2. The bottom layer of LinkedList uses a two-way linked list. This data structure stores data. Linked lists are suitable for frequent additions and deletions of elements, but are not suitable for query operations. 3. The bottom layer of Vector is the same as the ArrrayList collection, but Vector is thread-safe and less efficient, so it is rarely used now. 4. Map collection inheritance…
[Reprint] Detailed explanation of the relationship between ArrayList, List, LinkedList, and Collection in java
Reprint link: http://www.cnblogs.com/liqiu/p/3302607.html Detailed explanation of the relationship between ArrayList, List, LinkedList, and Collection in java 1. Basic introduction (Set, List, Map) Set (set): The elements in the set are not ordered in a particular way, and there are no duplicate objects. Some of its implementation classes can sort objects in a collection in a specific way. List (list): The elements in the collection are sorted by index position. There can be duplicate objects, allowing objects to be retrieved according to their index position in the collection. Map (mapping): Each element in the collection contains a pair of key objects and value objects. There are no duplicate key objects in the collection, and the value objects can be repeated. Some of its implementation classes can sort key objects in a collection. 2. Basic interfaces and types 1. Iterator interface This interface allows traversing all elements in the collection. There are three methods: public boolean hasNext(): Determine whether there is a next element. public Object next(): Get the next element. Note that the return value is Object and may require type conversion. If there are no more elements available, a NoSuchElementException is thrown. Before using this method, you must first use…
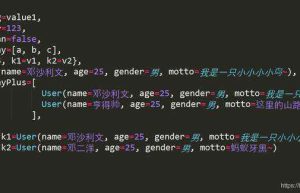
Implementation of placing arrays in java configuration files_@ConfigurationProperties binding configuration information to Array, List, Map, and Bean…
Related instructions: In SpringBoot,We can obtain and bind the information in the configuration file in the following ways: @Value annotation. Use Environment. @ConfigurationProperties annotation. By implementing the ApplicationListener interface ,registering the listener, for hard-coded acquisition, please refer to:https://www.jb51.net/article/187407. htm Hard coded loading file acquisition. …… Note : Under normal circumstances, the first and second methods are enough; but if you want to get them directly from the configuration file As for arrays, lists, maps, and objects, the support for the first and second types is not very good. Currently, only arrays, lists, and maps are available. As for beans, I’m a little helpless. At this time we can use the third method to obtain. Environment Description:Windows10, IntelliJ IDEA, SpringBoot 2.1.5.RELEASE @ConfigurationProperties annotation to obtain configuration information and bind to the object example: ; Preparation work:Introducing spring-boot-configuration-processor dependency Give me my complete pom.xml file: xsi:schemaLocation=”http: //maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd”> 4.0.0 org.springframework.boot spring-boot-starter-parent 2.1.5.RELEASE com.aspire.yaml-properties yaml-properties 0.0.1-SNAPSHOT Yaml file and properties file syntax examples yaml file and properties file syntax examples 1.8 org.springframework.boot spring-boot-starter org.projectlombok lombok true org.springframework.boot spring-boot-starter-test test org.springframework.boot spring-boot-configuration-processor org.springframework.boot spring-boot-maven-plugin Load demo. The information in the properties file is bound to the bean, and injected into the container example: Project…
Java8ListtoMap<String,Map<String,List>>
I have the following courses: public class Foo { private String areaName; private String objectName; private String lineName; } Now I want to convert List to Map<String, Map<String, List>>. I found this answer helped me develop the following Code: Map<String, Map<String, List>> testMap = foos.stream().collect(Collectors.groupingBy(e -> e.getAreaName(), Collectors.groupingBy(e -> e.getObjectName(), Collectors.collectingAndThen(Collectors.toList(), e -> e.stream().map(f -> f.getLineName()))))); The only problem with this code is the part that it should convert to List. I can’t find the part that converts Foo to List method. Another approach results in Map<String, Map<String, List>>: Map>> testMap = ventures.stream().collect(Collectors.groupingBy(e -> e.getAreaName(), Collectors.groupingBy(e -> e.getObjectName(), Collectors.toList()))); What do I need to do to get the changes to Map<String, Map<String, List>> from List? 1> Eran..: In order to obtain List and Stream element types are different types, you should link the Collectors.mapping collector to groupingBy: Map<String, Map<String, List>> testMap = foos.stream() .collect(Collectors.groupingBy(Foo::getAreaName, Collectors.groupingBy(Foo::getObjectName, Collectors.mapping(Foo::getLineName, Collectors.toList()))));
Java streams are grouped by List<Map> into Map<Integer,List>
I have one List<Map> From Spring NamedParameterJdbcTemplate queryForList call. The data returned is as follows: [{“id”:5,”uid”:6}, {“id”:5,”uid”:7}, {“id”:6,”uid” :8}, {“id”:7,”uid”:7}, {“id”:8,”uid”:7}, {“id”:8,”uid”:9}] How can I rearrange my data in the following format? {5:[6, 7], 6:[8], 7:[7], 8:[7, 9]} I want to get back a Map<Integer, List> Anyone knows how to achieve this? Any help is greatly appreciated? 1> Eugene..: This is a Collectors.groupingBy job for downstream collectors Collectors.mapping Map<Integer, List> result = l.stream() .collect(Collectors.groupingBy( m -> (Integer) (m.get(“id”)), Collectors.mapping(m -> (Integer) m.get(“uuid”), Collectors.toList()))); Or no stream at all: list.forEach(x -> { Integer key = (Integer) x.get(“id”); Integer value = (Integer) x.get(“uuid”); result.computeIfAbsent(key, ignoreMe -> new ArrayList()).add(value); });
Java8 converts Map<K,List> to Map<V,List>
I need to convert Map<K, List> to Map<V, List>. I have been struggling with this for a while Question. Obviously how to convert Map to Map<V, List>: .collect(Collectors.groupingBy( Map.Entry::getKey, Collectors.mapping(Map.Entry::getValue, toList()) ) But I can’t find a solution to the original problem. Is there some easy to use java-8 way to do this? 1> Eugene..: I think you are close, you need flatMap those entriesStream and collect from there. I’ve used the already existing SimpleEntry, but you could also use Pair of some type. initialMap.entrySet() .stream() .flatMap(entry -> entry.getValue().stream().map(v -> new SimpleEntry(entry.getKey(), v))) .collect(Collectors.groupingBy( Entry::getValue, Collectors.mapping(Entry::getKey, Collectors.toList()) )); Well, if you don’t want the extra overhead of creating these SimpleEntry instances, you can do something different: Map<Integer, List> result = new HashMap(); initialMap.forEach((key, values) -> { values.forEach(value -> result.computeIfAbsent(value, x -> new ArrayList()).add(key)); }); I was just writing an answer (tried it on my IDE first) but it didn’t pass compilation. Then you posted an answer that looked the same as mine, but your answer did pass assembly. I couldn’t find out Differences, until I compared them in the comparison tool and discovered that I invented a new class – “SimplyEntry”… +1
Java8 stream – Find the maximum count value of Map<String,List>
I’m new to Java 8 and wanted to see if I could find the same thing as the code below using streams. The code below basically tries to find the key with the maximum number of values and return that key. I can’t find much help anywhere in this format. int max = 0; String maxValuesString = null; for (Map.Entry<String, List> entry : map.entrySet()) { if(max <entry.getValue().size()) { maxValuesString = entry.getKey(); max = entry.getValue().size(); } } ernest_k.. 8 You can use the max comparator to check the size of a value String maxValuesString = map.entrySet() .stream() .max(Comparator.comparingInt(entry -> entry.getValue().size())) .map(Map.Entry::getKey) .orElse(null); Edit: Thanks to Andrés for the clean comment below optional.map 1> ernest_k..: You can use the max comparator to check the size of a value String maxValuesString = map.entrySet() .stream() .max(Comparator.comparingInt(entry -> entry.getValue().size())) .map(Map.Entry::getKey) .orElse(null); Edit: Thanks to Andres for the clean comment below optional.map Best answer: 1) The name variable is the same as the question. 2) If map is empty, don’t crash and burn, i.e. it should have the same result as question(`null`). Use `.map(Map. Entry::getKey).orElse(null)` replaces `.get().getKey()` to support null mapping.
java iterative map<string, list, getordefault method
I have a map of <string, List >, what I want to do is if the list exists then add a string to map the value, else create a list and add it to the list and insert map. s1, s2 are strings. Code: Map<String, List> map = new HashMap(); map.put(s1,(map.getOrDefault(s1, new LinkedList())).add(s2)); Error: error: incompatible types: boolean cannot be converted to List What’s wrong with this!!! 1> janith1024..: Add list ‘map.getOrDefault(s1,new LinkedList ())’s method .add(s2)’ returns a Boolean value, so you must execute it in a separate line So try this Map<String, List> map = new HashMap(); List list = map.get(s1); if(list == null){ list = new LinkedList(); map.put(s1,list); } list.add(s2); If using java 8 and need to do something like this in a single line map.computeIfAbsent(s1, k -> new LinkedList()).add(s2);
Java8 convert List to Map<Integer,List>
I have a List object and want to convert it Map<Integer, List> into a map key representing a person’s property level .It may be possible to have multiple Person objects with the same rank in the source list, in which case I want to group them all into a List, corresponding to the corresponding rank in the generated Map. So far I have the following code public class PersonMain { public static void main(String[] args) { Person person1 = new Person(); person1.setName(“person1”); person1.setGrade(1); Person person2 = new Person(); person2.setName(“person2”); person2.setGrade(2); Person person3 = new Person(); person3.setName(“person3”); person3.setGrade(1); List persOns= Arrays.asList(person1, person2, person3); Map<Integer, List> persOnsMap= persons.stream() .collect(Collectors.toMap(Person::getGrade, PersonMain::getPerson, PersonMain::combinePerson)); System.out.println(personsMap); } private static List getPerson(Person p) { List persOns= new ArrayList(); persons.add(p); return persons; } private static List combinePerson(List oldVal, List newVal) { oldVal.addAll(newVal); return oldVal; } } Is there a better way to achieve this? 1> Ousmane D…: Your current solution is good, but using the groupingBy collector is more intuitive: Map<Integer, List> persOnsMap= persons.stream() .collect(Collectors.groupingBy(Person::getGrade)); This overload of the groupingBy collector is very simple as it only requires a classifier (Person::getGrade) function which will extract the key Group objects of a stream.
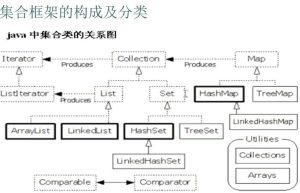
Dark Horse Programmer – Java Basic Collection (1) Collection, set, list
——Java training, Android training, iOS training, .Net training, looking forward to communicating with you! —— /strong> There are many collections in Java, also called containers. The following figure shows the composition and classification of the collection framework. 1. Why do collection classes appear? Object-oriented languages embody things in the form of objects, so in order to facilitate the operation of multiple objects, objects are stored. Collections are the most commonly used way to store objects. 2. Arrays and collections are both containers. What are the differences? Although arrays can also store objects, their length is fixed; collection lengths are variable. Basic data types can be stored in arrays, while collections can only store objects. 3. Characteristics of collection classes Collections are only used to store objects. The length of the collection is variable, and the collection can store different types of objects. Collection Collection is a common interface in the collection framework. There are two sub-interfaces under it: List and Set. Affiliation: Collection |–List//Elements are ordered and elements can be repeated. Because the collection system has indexes. |–Set//The elements are unordered and the elements cannot be repeated. The following is an overview of Collection: 1 import java.util.*; 2…