java, useful code snippets
In the process of writing programs, we often encounter some common functions. These functions or effects are often similar, and the solutions are also similar. Below are some useful code snippets that I picked up while writing the code. 1. When operating the same Collection in a multi-threaded environment, thread synchronization problems will occur, and exceptions may even be thrown sometimes Solution: Use Collections.synchronizeMap() and use the following code to access or delete elements 1 public class ConcurrentMap { 2 private Map map = Collections.synchronizedMap(new HashMap()); 3 4 public synchronized void add(String key, String value) { 5 map.put(key, value); 6 } 7 8 public synchronized void remove(String key) { 9 Set<Map.Entry> entries = map.entrySet(); 10 Iterator<Map.Entry> it = entries.iterator(); 11 while (it.hasNext()) { 12 Map.Entry entry = it.next(); 13 if (key.equals(entry.getKey())) { 14 it.remove(); 15 } 16 } 17 } 18 19 public synchronized void remove2(String key) { 20 Set<Map.Entry> entries = map.entrySet(); 21 entries.removeIf(entry -> key.equals(entry.getKey())); 22 } 23 } View Code 2. Obtain IP according to URL Solution, use java.net.InetAddress tool class /** * Get the corresponding IP based on the url * @throws UnknownHostException UnknownHostException */ @Test public void testGetIP() throws UnknownHostException { Pattern domainPattern = Pattern.compile(“(?<=://)[a-zA-Z\\.0-9]+(?=\\/)");…

(Repost) Random numbers are deceiving, .Net, Java, and C bear witness for me
(Reprinted from: http://www.cnblogs.com/rupeng/p/3723018.html ) Almost all programming languages provide a method of “generating a random number”, that is, calling this method will generate a number, and we do not know in advance what number it will generate. For example, write the following code in .Net: Random rand = newRandom(); Console.WriteLine(rand.Next()); The results after running are as follows: The Next() method is used to return a random number. The results of your execution of the same code may be different from mine, and the results of my multiple runs may also be different. These are random numbers. 1. Trap It seems to be a very simple thing, but there are pitfalls when using it. I wrote the following code to generate 100 random numbers: for(int i=0;i<100;i++) { Random rand = new Random(); Console.WriteLine(rand.Next()); } It’s so strange. There are many consecutive and identical “random numbers” generated. What kind of “random numbers” are these? Someone pointed out that “just put new Random()” outside the for loop: Random rand = newRandom(); for(int i=0;i<100;i++) { Console.WriteLine(rand.Next()); } Run results: It is indeed possible! 2. Why is this? This starts from the principle of “random number” generation in computers. We know that computers are very…
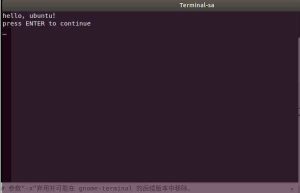
Configure gedit as the most powerful compilation tool (C, C++, Java)
Enter root mode 1 sudo -i Open gedit 1 gedit &# 160; In the preferences, you can choose the color theme, line number, etc. of the text editor Please complete this operation before proceeding to the next step dconf-editor Enter the last item of the terminal in plugins, cancel use-them-colors, if gedit is not installed -plugins Execute sudo apt-get install gedit-plugins Then we proceed to the next step, Write Sell to compile and run the code in gedit Click on the external management tool Only this content, let’s click the plus sign in the lower left corner Create the first tool: Compile #The name can be arbitrary #!/bin/shfullname=$GEDIT_CURRENT_DOCUMENT_NAMEname=`echo $fullname | cut -d. -f1` suffix=`echo $fullname | cut -d. -f2`if [ $suffix = “c” ]; then gcc $fullname -o $name -O2 -Wall -std=gnu99 -static -lm elif [ $suffix = “cpp” ] || [ $suffix = “c++” ] || [ $suffix = “cc” ] || [ $suffix = “cxx” ] || [ $suffix = “C ” ]; then g++ $fullname -o $name -O2 -Wall -std=gnu++0x -static -lmelif [ $suffix = “java” ]; then javac $fullname – encoding UTF-8 -sourcepath . -d .fi View Code Copy these…
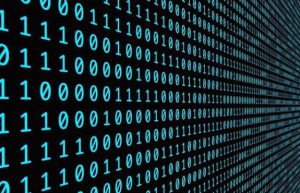
Look at performance optimization from several performance test cases in three languages (C++, Java, C#)
With the development of time, the current virtual machine technology is becoming more and more mature. In some cases, the performance of intensive computing of virtual machines such as Java and .Net is similar to that of C++. In some cases, it is even better. excellent. This article analyzes several performance test cases in detail and explores the reasons behind the phenomenon. Let’s look at two simple test cases. As shown in the figure below, they all loop 5000 times, operate the continuous memory of len = 1000000, and calculate the execution time. The left side is test1 and the right side is test2. Similar programs were tested under .net core 3.0 Preview6. The test results are compared as follows: We can see that for test1, the C++ version is much faster. For test2, the performance of the C# version and the C++ version are equivalent, or even slightly faster. Why does this happen? Let’s analyze it in detail: The assignment of the loop of test1 is position-independent, so the compiler can optimize it through parallel computing instructions such as SIMD. The assignment of the loop of test2 is position-related, and it is difficult for the compiler to optimize using…
[Original] Highlight your code to show off your level [With source code, supports highlighting in most common languages, such as C#, JAVA, etc.]
Let everyone see the intuitive effect first: [Project Structure Chart] [Highlighted code diagram (default is C#, readers can modify it to other languages according to comments)] [Rendering (1)] 【Rendering (2)】 This article was originally intended to be named the continuation of “File-driven website development”, but after thinking about it, it would be clearer to list the title separately, so the current name is used. For the article on file-driven website development, please refer to:http://www.cnblogs.com/OceanChen/archive/2009/02/04/1383794.html When there is no highlighting method, we often write the styles ourselves. Of course, we can also directly use Microsoft Word to convert it into HTML, but the converted HTML is very redundant. Actually, this is not a sophisticated technology. It is just implemented using the [SyntaxHighlighter 2.0] library provided by Google. If you want to download [SyntaxHighlighter 2.0], please refer to the following address: http://code.google.com/p/ syntaxhighlighter/ I wrote this series of articles mainly to consider this scenario: If you have rented a virtual server, you will definitely encounter this problem. It is only for the database, and you need to pay extra, and the price is very high! It may be higher than the price of your virtual server! This is also one of the…
“Don’t think that knowing C++, Java, and Net means you’re awesome.” This article is in tandem with an issue of Langyan Finance that I saw.
Original repost address: http://topic.csdn.net/u/20111103/08/68a3afa2-ec55-4236-aa9d-d6428a363499.html Video address: http://video.sina.com.cn/v/b/64293312-1092672395.html I recently saw an article on the Jiaotong University Siyuan Forum (http://bbs.xjtu.edu.cn/BMYALNSNYULXCXXLQAAUHKMUVFHBKJDLILPS_B/). It seems that what everyone is complaining about is not a technical issue, but a systemic issue and an economic issue. . I don’t know if the author of this article is born in the 1980s. Seeing everyone’s confusion, I feel that this is a historical problem encountered by a generation. Our economic model has caused such problems in society, and then caused everyone’s complaints. Social classes are solidified, and those who have already made profits in society only seek immediate benefits and do not seek innovation; the cost of starting a business for socially dynamic groups is too high, and they finally choose to return to the original backward system. We are working as pure wage earners for Europe and the United States. The so-called business owners in China are exploited by Europe and the United States, and the Chinese are exploited by business owners. Facing history and reality, we can only maintain a positive attitude and do some self-awareness. The transformation of a nation’s consciousness will not be completed in an instant, nor will it be painless. Let’s see…

go, java, netcore performance test records
Test logic: Loop 100,000 times to output and print hello world Test platform: windows Test results Language Memory occupied Packet size Time consuming go 5324k Executable file: 2172k Average 8502 milliseconds java 175326k Bytecode file: 2k Average 8537 milliseconds dotnetcore 3612k Packaging and publishing: 65.4m Average 8371 milliseconds Screenshot introduction go: Run main.exe directly Code: package mainimport ( “fmt” “os” ” os/signal” “syscall” “time”)func main() { t1 := time.Now() // get current time //logic handlers for i := 0; i <100000; i++ { fmt.Println(“hello world”) } elapsed := time.Since(t1) fmt.Println (“App elapsed: “, elapsed) sig := make(chan os.Signal, 2) signal.Notify(sig, syscall.SIGTERM, syscall.SIGINT) <-sig } Directory after release java: Run the compiled bytecode file java HelloWorldCode import java.io.IOException;public class HelloWorld { public static void main(final String[] args) throws IOException { final long time1 = System.currentTimeMillis(); int i = 0; for (; i <100000; i++) { System. out.println(“hello world”); } final long time2 = System.currentTimeMillis(); System.out.println(“The current program takes time:” + (time2 – time1) + “ms”); System.in.read(); }} Compiled directory dotnetcore: Run netcore_prj.exe directlycode using System ;using System.Diagnostics;using System.Threading.Tasks;using System.Threading;namespace netcore_prj{ class Program { static async Task Main(string[] args) { var watch = new Stopwatch(); watch.Start(); for (var i = 0;…

JAVA Haoge teaches, Lanou’s three major courses python, Java, and HTML5 are started at the same time, so you can learn in a hurry
Multiple courses are offered simultaneously 16 July 2018 The sun is blazing in July , Just like the lively atmosphere of the Blue Ou class opening, today there are three classes of H5,Java,Python at the same time The class has started! In fact, during the two days on the weekend, all the friends of Lanou were working overtime to prepare for the new students. The students came and were directly arranged to the prepared dormitories and classrooms for the opening of the class. All the items needed by the students were arranged one by one, just for the smooth start of the class today. Campus General Manager Hao Ge extended a warm welcome to all students and briefly introduced the development of Lanou, the status of the industry, and the direction and planning of learning. In his speech, he expressed that “the next 4 months of study and life” are 10.5 hours of high-intensity study every day. “Although it is very tiring,” you will feel sincerely when you graduate and get a high-paying offer. Gratitude – Then you will thank yourself for working hard at this moment. The best gift that Lanou gives to students is all-round meticulous arrangements to…

java, export excel, control data traversal vertically or horizontally, use easyExcel
java,Export excel,Control data traversal vertically or horizontally java export excel,Use easyExcel, export in the specified format,Export by template,Custom header export The premise here is that “you use easyExcel” even if you don’t use it, you can try it “it’s very simple.” If you find it useful, remember to follow it for free,Like,Collect it in three lines public void exportExcelByEasy(HttpServletResponse response, List<?> importlist) throws IOException {//1,Find the template file, and convert it into an input stream&# xff1a;templateFileInputStream;InputStream templateFileInputStream = this.getClass().getClassLoader().getResourceAsStream( span>”com/exceltemplate/testttt.xlsx”);//2,outputStream:The output stream of the file to be exported;OutputStream outputStream = response.getOutputStream();ExcelWriterBuilder excelWriterBuilder &# 61; EasyExcel.write(outputStream).withTemplate( templateFileInputStream);ExcelWriter excelWriter = excelWriterBuilder.build();//settings Is the list horizontal or vertical —–The default is vertical,HORIZONTAL means horizontal filling FillConfig fillConfig = FillConfig.builder().direction(WriteDirectionEnum.HORIZONTAL).build();try {WriteSheet writeSheet = EasyExcel.writerSheet().build();//3,Fill data into the sheet:excelWriter.fill(new FillWrapper (“data1”, importlist), writeSheet);excelWriter.fill(new FillWrapper( “data2”, importlist), fillConfig, writeSheet) ;//4,Set the output stream format and file name:response.setContentType(“application/vnd.openxmlformats-officedocument.spreadsheetml.sheet”);response.setCharacterEncoding(“utf-8”);response.setHeader(“Content-disposition” , “attachment;filename*=utf-8''” + “test” + “.xlsx”);} finally {//5,Finally,Don’t forget to finish, ;Close IO stream;if (excelWriter != ; null) {excelWriter.finish();}}} In fact, the key point is here,You can just configure it like this, Why do we need to add data1 and data2? is actually to distinguish different data sets. Of course, you can also use different prefixes for the same…