Add, delete, modify and query pymongo operations
Module import from pymongo import MongoClient Instantiate client and establish connection client=MongoClient(host=”127.0.0.1″,port=27017) collection=client[“test”][“std “] #test is the database, std is the collection Insert a piece of data collection.insert({“name”:”xiaoming”,”age”:18}) Insert a piece of data and modify the default _id value. collection.insert({“_id”:20181126,”name”:”xiaoming”,”age”:18}) Insert a piece of data, use ret to receive it, and print out the value of _id ret=collection.insert({“_id”:20181126,”name”:”xiaoming”,”age”:18}) print(ret) #Run result: 20181126 Insert multiple pieces of data insert_many(), data_list=[{“name”:”test{} “.format(i)} for i in range(10)] collection.insert_many(data_list) Query a record find_one() t=collection.find_one({“name”:”xiaowang”}) print(t) To query multiple records find(), the output is a cursor. t=collection.find({“name”:”xiaowang”}) print(t) Update a piece of data update_one() collection.update_one({“name”:”xiaoming”},{“$set”:{“name”:”xiaozhang”}}) Update multiple pieces of data update_many() collection.update_many({“name”:”xiaoming”},{“$set”:{“name”:”xiaozhang”}}) delete_one() to delete a piece of data collection.delete_one({“name”:”xiaowang”}) Delete multiple pieces of data delete_many() collection.delete_many({“name”:”xiaowang”})
Add, delete, modify and query django database tables
1. Add table records There are two methods for a single table # Two ways to add data # Method 1: The instantiated object is a table record Frank_obj = models.Student(name =”Haidong”,course=”python”,birth=”2000-9-9″,fenshu=80) Frank_obj.save() # Method 2: models.Student.objects.create(name =”Haiyan”,course=”python”,birth=”1995-5-9″,fenshu=88) 2. Query table records Query related API # Query related API # 1. all(): View all student_obj = models.Student.objects.all() print(student_obj) #The printed result is a QuerySet collection # 2. filter(): The AND relationship can be implemented, but the OR relationship needs to be implemented with the help of Q query. . . # No error will be reported when it cannot be found print(models.Student.objects.filter(name=”Frank”)) #View names whose names are Frank print(models.Student.objects.filter(name=”Frank”,fenshu=80)) #View those whose name is Frank and whose score is 80 # 3. get(): If it cannot be found, it will report an error. If there are multiple values, it will also report an error. You can only get the one with one value print(models.Student.objects.get(name=”Frank”)) #What you get is the model object print(models.Student.objects.get(nid=2)) #What you get is the model object # 4. exclude(): exclusion conditions print( models.Student.objects.exclude(name=”Haidong”)) # View information except that the name is Haidong # 5. values(): It is a method of QuerySet (convert the object into the form…
Nodejs operates mongodb, add, delete, modify and check
I haven’t learned node for a long time. I read half of the book before. Today I continued to learn and found that the version problem is very annoying. There are a lot of errors when executing the examples in the book. Want to learn nodejs to operate db, spend half a day on Baidu, write a bunch of sb for fun? Simply write a lot of things and it won’t work. If you need code, you also need to read it written by others, and it is still necessary to write a note. 1. Install mongodb, http://www.mongodb.org/. Now that .msi is available, you can install it without a command, but by default it will be in the search in the lower left corner after installation. Not found. The default path is in the c drive C:\Program Files\MongoDB 2.6 Standard\bin 2. To run mongodb, first start the mongo service and go to the mongo bin directory. This may be the first time You need to create a dbpath (you can also specify it yourself mongod –dbpath XXXX/data), md datamd data\ db 3. Connect to mongoDon’t close the window just now, and open another cmd The default link is to the…
Add, delete, modify and query djangomysql table
1. Add data Call this routeExecute the processing method of ModelsCaozuo The first instantiation class class ModelsCaozuo(View): ‘‘‘ Database addition, deletion, modification and query ‘‘‘ def get(self, request): article = Article() article.title = “How to add data to a table that has multiple fields” article.content = “Instantiate model and assign value through .attr” article.message = “Use django’s save persistent data” article.save() return HttpResponse(“%s%s%s” % (article.title, article.content, article.message)) The second type is not instantiated class ModelsCaozuo1(View): ‘‘‘ Database addition, deletion, modification and query ‘‘‘ def get(self, request): Article( title=”Add data without instantiation, use the class directly”, content=”66666666666″, message = “Also persist data through django’s save” ).save() return HttpResponse(“%s%s%s” % (Article.title, Article.content, Article.message)) # Can’t get data? 2. Query data Query all data in the Article table Article.object.all() ps: Return multiple pieces of data (list collection of Article instances, for in) class ModelsCaozuo2(View): ‘‘‘ Query all data in the Article table‘‘‘ def get(self, request): content_all = Article.objects.all() print content_all # List of QuerySet instances available for in # <QuerySet [, ] return render(request, ‘mysql_select.html‘, locals()) Mysql_select.html page gets attributes <body> {% for con in content_all %} Each piece of data in the table corresponds to an instance con of the Article class…

Add, delete, modify and check gomap
package main import “fmt” func main() { //Definition map a := make(map[int]string) //Add a[20190902] = “abc” a[20190903] = “cde” fmt.Println(a) //Modify a[20190902] = “aaa” fmt.Println(a) //Delete delete(a, 20190902) fmt.Println(a) //Search fmt.Println(a[20190903]) }
Code for using javascript to operate ACCESS database (read, add, modify and delete) under static pages
The static page reads the ACCESS database.htm The code is as follows: Add data to the database page-Insert.htm The code is as follows: friend_name friend_nickname Delete function-Delete.htm Code As follows: Modify functionUpdata.htm The code is as follows:
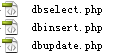
Add, delete, modify and check the interaction between php and javascript in php database
Using language to operate a database is a top priority. If you don’t know how to operate a database in a language, then you still haven’t learned the language. How to operate database with PHP is not difficult At the same time, the value of php can also be controlled with Javascript script Generally, the value of PHP is passed to Javascript, and the reverse operation is generally not done 1. Basic objectives First of all, there is a user information table user in mysql. The fields in it are id, username and password. Open the web page dbselect.php, and first use php to find out the entire user table: Then, insert the data column, enter the data, and the data can be inserted into the user table in mysql In the column for modifying data, the first drop-down menu is created through Javascript, and the number of drop-down options is given based on the amount of data in the table. The second drop-down menu allows the user to select the column to be modified The third input box allows the user to enter the value to be modified As for why the data is not deleted, it is because…
Add, delete and copy java files and directories
When developing using Java, methods such as adding, deleting, and copying files and directories are often used. I wrote a widget class. Share it with everyone and hope you can correct me: package com.wangpeng.util; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.FileWriter; import java.io.InputStream; import java.io.PrintWriter; /** * @author wangpeng * */ public class ToolOfFile { /** * Create a directory * * @param folderPath * Table of contents * @throwsException */ public static void newFolder(String folderPath) throws Exception { try { java.io.File myFolder = new java.io.File(folderPath); if (!myFolder.exists()) { myFolder.mkdir(); } } catch (Exception e) { throw e; } } /** * Create a file * * @param filePath * file path * @throwsException */ public static void newFile(String filePath) throws Exception { try { File myFile = new File(filePath); if (!myFile.exists()) { myFile.createNewFile(); } } catch (Exception e) { throw e; } } /** * Create file and write content * * @param filePath * file path * @param fileContent * The content of the file being written * @throwsException */ public static void newFile(String filePath, String fileContent) throws Exception { // Convenience class for writing character files FileWriter fileWriter = null; // Print a formatted representation of…
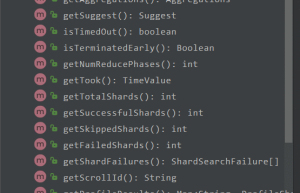
Add, delete, modify and check_ESJavaAPI’s add, delete, modify and check
After basically understanding and operating Elasticsearch’s query syntax in the Kibana Dev Tools console, it’s time to use ES in actual applications. So how to use ES in Java? There are several ways to achieve it: Transport Client Java High Level REST Client Spring Data Elasticsearch TransportClient can be used for older versions of Elasticsearch; New version recommendations Use Java High Level REST Client (backwards compatible); Of course, you can also use Spring Data Elasticsearch provided by Spring Data. This article will only introduce the Transport ClientAPI, and then slowly introduce REST Client and Spring Data Elasticsearch. Transport Client NOTE :In 7.0.0 Deprecated. TransportClient has been deprecated in favor of Java High Level REST Client and will be removed in Elasticsearch 8.0. Elasticsearch version 8.x will remove TransportClient. Why do we need to introduce its API here? After all, some veterans are still using the previous version. And the API is similar in many places. Then learn more. /p> Introduce jar package org.elasticsearch.clientgroupId> transportartifactId> 7.9.0version>dependency> Note that the version number must be consistent with the version number of the Elasticsearch you installed. Create a connection to ES You must first connect to ES to perform a series of API test…